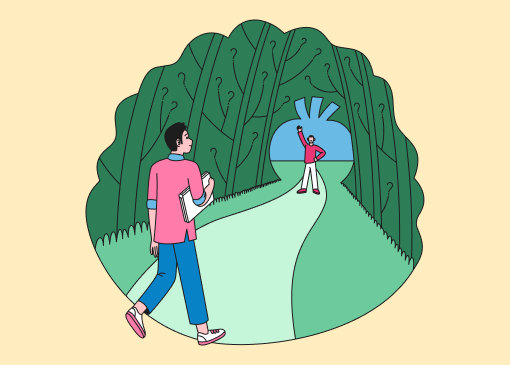
13 Key Android Interview Questions (and Answers) for Hiring a Senior Developer
Contents
Hiring a senior Android developer is a crucial decision that can significantly impact your projects and business success. With Android holding a dominant 71.44% share of the global mobile operating system market, identifying the right talent is essential to effectively leverage this vast market.
This guide equips you with the key Android interview questions to ask during the interview process. By focusing on essential areas, you’ll be able to pinpoint top candidates who can ensure the robustness of your Android applications. Let’s streamline your hiring process and find the right fit for your team.
Topics to Raise During the Interview with an Android Engineer
To thoroughly evaluate a senior Android developer, it’s essential to delve into critical areas such as object-oriented design, reactive programming, Java and Kotlin expertise, and Android views. By focusing on the following Android interview topics, you can gain a comprehensive understanding of the candidate’s technical proficiency and practical experience.
Object-Oriented Programming (OOP) & Object-Oriented Design (OOD)
Understanding and applying OOD principles is crucial for designing scalable and maintainable software. Senior candidates should demonstrate a deep understanding of creating proper abstractions and ensuring program scalability and flexibility through techniques like high cohesion and loose coupling. Their experience should reflect the ability to design systems that are easy to extend and modify without causing a ripple effect of changes.
Reactive Programming
Reactive programming is essential for building responsive and efficient Android applications, especially those that handle asynchronous data streams. A senior developer should be proficient with frameworks like RxJava or Kotlin Coroutines and understand concepts like observables, subscribers, flow, and the reactive streams API. This knowledge ensures they can handle complex asynchronous tasks seamlessly.
Java
Java remains a cornerstone of Android development. Senior developers should have a strong foundation in Java, including deep knowledge of generics (covariance & contravariance, wildcards, type erasure), the Java Memory Model (JMM), and concurrency. Understanding these concepts allows developers to write flexible, type-safe, and efficient code and to manage concurrent operations safely and effectively.
Kotlin
Kotlin is the preferred language for modern Android development due to its concise syntax, null safety, and advanced features. Senior developers should be proficient in Kotlin and understand advanced concepts like extension functions, coroutines, higher-order functions, and Kotlin-specific design patterns. This knowledge allows them to write more efficient, expressive, and maintainable code.
Android Views
Understanding Android Views is crucial for creating user interfaces. Senior developers should have deep knowledge of the Android View hierarchy, custom views, view binding, and performance optimization techniques. It ensures they can create responsive, efficient, and user-friendly interfaces that enhance the overall user experience.
Unit Testing
Unit testing is critical for maintaining code quality and ensuring reliability. Senior developers should have solid experience with testing frameworks like JUnit and Espresso. They should understand how to write testable code, mock dependencies, and set up CI/CD pipelines for automated testing. They should also address common issues with fragile tests by writing robust, maintainable tests and providing solutions for test brittleness and flakiness.
Inversion of Control (IoC) and Dependency Injection (DI)
IoC and DI are design patterns that enhance the modularity and testability of code. Senior developers should be familiar with IoC principles and frameworks like Dagger or Hilt. This knowledge helps in creating loosely coupled components, making the codebase easier to manage, extend, and test. They should understand how to apply these principles to create a flexible and maintainable architecture.
Team Organization and Process Establishment
Senior developers often play a key role in organizing teams and establishing processes. They should understand how to structure development teams to maximize productivity and establish best practices to ensure high-quality code and minimize bugs. It involves setting up efficient workflows, conducting regular code reviews, and fostering a collaborative environment.
Continuous Delivery (CD)
Setting up a CD process is critical for delivering updates rapidly and reliably. Senior developers should be experienced in configuring CD pipelines that deploy builds to distribution platforms automatically. This includes ensuring the build process integrates smoothly with version control and follows a well-defined branching model, such as GitFlow.
In-Depth Android Dev Interview Questions
To effectively evaluate a senior Android developer’s proficiency, consider these comprehensive Android interview questions designed to assess their technical knowledge, coding skills, and real-world experience.
- Explain how you apply object-oriented design principles in your Android projects. Can you provide specific examples of how you ensure high cohesion and loose coupling?
Good Answer: The candidate should discuss their approach to designing systems with high cohesion and loose coupling, such as using interfaces and abstract classes for flexibility, adhering to SOLID principles, and ensuring each class has a single responsibility. Examples include using dependency injection to decouple class dependencies or designing a modular architecture that allows independent component development.
Red Flag: Inability to explain OOD principles clearly or provide concrete examples from their experience, indicating a lack of depth in design thinking.
- Describe your experience with reactive programming in Android. How have you used frameworks like RxJava or Kotlin Coroutines?
Good Answer: The candidate should explain their experience with RxJava or Kotlin Coroutines, including handling asynchronous data streams, managing threading, and implementing reactive patterns. They should provide examples, such as using RxJava for network requests or Kotlin Coroutines for background processing.
Red Flag: Lack of familiarity with reactive programming frameworks or inability to provide practical examples.

- How proficient are you in Java, and how do you leverage Java in your Android development? Can you explain your understanding of advanced generics concepts like covariance, contravariance, and type erasure?
Good Answer: The candidate should demonstrate strong Java skills and discuss their experience with Java syntax, libraries, and design patterns. They should also explain advanced generics concepts and provide examples of using them to create flexible and type-safe code. Examples might include creating reusable library components or designing a robust API.
Red Flag: Limited knowledge of Java or inability to work with advanced generics concepts.
- What are the advantages of using Kotlin over Java in Android development? Can you provide examples of Kotlin-specific features you have used?
Good Answer: The candidate should discuss Kotlin’s benefits, such as null safety, extension functions, and coroutines. They should provide examples, like using extension functions to add functionality to existing classes or coroutines for asynchronous programming.
Red Flag: Lack of experience with Kotlin or inability to articulate its advantages over Java.
Can you explain the Android View hierarchy and describe a complex UI you have built, including any custom views?
Good Answer: The candidate should explain the Android View hierarchy and discuss their experience building complex UIs, including the use of custom views, view binding, and performance optimizations. They should provide specific examples of challenging UI projects.
Red Flag: Inability to explain the View hierarchy or lack of experience with custom views and performance optimization.
- How do you approach unit testing in your Android projects? Can you give examples of testing frameworks and tools you have used?
Good Answer: The candidate should discuss their experience with unit testing, mentioning frameworks like JUnit and Espresso and mocking tools like Mockito. They should provide examples of writing test cases, ensuring code coverage, and integrating tests into CI/CD pipelines. They should also discuss strategies for addressing fragile tests and ensuring test reliability.
Red Flag: Lack of experience with unit testing frameworks or inability to provide examples of writing and running tests.
- Describe a situation where you used inversion of control (IoC) and dependency injection (DI) in an Android project. What were the benefits?
Good Answer: The candidate should explain their experience with IoC and DI, mentioning frameworks like Dagger or Hilt. They should provide examples, such as using DI to manage dependencies in a large project, leading to better modularity and testability. They might discuss how DI facilitated testing by allowing the injection of mock dependencies.
Red Flag: Lack of understanding of IoC and DI principles or inability to provide practical examples.
- Scenario: Your app is experiencing performance issues on low-end devices. How would you diagnose and address these issues?
Good Answer: The candidate should mention using profiling tools like Android Profiler, analyzing logs, optimizing resource usage (e.g., reducing image sizes, optimizing layouts), minimizing memory usage, and considering lazy loading or other performance improvements.
Red Flag: No structured approach to diagnosing performance issues or lack of familiarity with profiling tools.
- Scenario: You need to integrate a significant new feature into an existing codebase without compromising stability. How do you approach this task?
Good Answer: The candidate should describe steps such as planning the integration, writing and running unit tests, conducting thorough code reviews, ensuring backward compatibility, and possibly using feature toggles for a gradual rollout.
Red Flag: Overlooking the importance of testing, code reviews, or backward compatibility.
- Scenario: An app crashes in production, but the issue cannot be reproduced locally. What steps would you take to resolve it?
Good Answer: The candidate should mention analyzing crash reports using tools like Firebase Crashlytics, gathering additional data from users, replicating the production environment locally, and applying targeted fixes. They should emphasize a systematic approach to debugging.
Red Flag: Lack of systematic debugging approach or unfamiliarity with crash reporting tools.
Evaluating Soft Skills and Team Leadership Capabilities
Assessing a senior Android developer’s soft skills and ability to lead is essential to ensure they can help structure development teams, establish efficient processes, and foster a collaborative environment.
- How do you foster collaboration and effective communication within a distributed team?
Good Answer: The candidate should explain their approach to fostering collaboration, such as using regular video meetings, collaborative tools (e.g., Slack, Jira, Confluence), and setting clear expectations for communication. They should provide an example, like organizing virtual team-building activities or establishing a buddy system for new team members. Highlighting their commitment to creating an inclusive and cohesive team environment is essential.
Red Flag: Lack of specific strategies or tools for fostering collaboration, inability to address challenges specific to distributed teams, or lack of prioritization of regular and clear communication.

- Can you provide an example of how you have supported junior developers on your team? What impact did it have?
Good Answer: The candidate should describe a mentoring experience, including specific actions like code reviews, pair programming, and providing feedback on projects. They should mention the positive impact on the junior developer’s growth and the overall team, such as improved code quality, increased productivity, or higher team morale. An example is setting up a structured mentorship program within the team.
Red Flag: No experience with supporting junior specialists, inability to demonstrate the impact of their mentoring efforts, lack of structured approach to mentorship.
- How do you contribute to continuous improvement and innovation within your team’s workflow and processes?
Good Answer: The candidate should discuss their approach to contributing to continuous improvement, such as suggesting regular retrospectives, proposing new ideas, and staying updated with industry best practices. They should provide an example, like telling an agile methodology that enhances team efficiency and productivity. Emphasizing their commitment to innovation and staying ahead in the field is important.
Red Flag: No strategies for continuous improvement, resistance to change or innovation, inability to provide specific examples or lack of engagement with industry trends.
- Describe a time when you supported your team through a significant change, such as adopting a new technology or process. How did you contribute to the transition?
Good Answer: The candidate should discuss a specific example of supporting their team through change, detailing their contributions, such as helping to communicate the benefits, addressing concerns, and providing assistance. They might describe their role in a transition to a new development framework, where they helped colleagues by sharing knowledge, creating documentation, or conducting informal training sessions.
Red Flag: Inability to provide a specific example, lack of strategies for supporting change, or failure to address team concerns and offer assistance.
Find the right senior Android Developer with Beetroot
Hiring a senior Android developer goes beyond assessing technical skills; it’s about discovering someone who truly fits your project’s aims and shares your company’s ethos. The questions we’ve shared aim to assist tech leads and CTOs in navigating this nuanced selection process, highlighting candidates who excel in coding and bring a unique perspective to your team.
At Beetroot, our goal is to connect your business with Android developers who are adept at answering tough Android app development interview questions and genuinely enthusiastic about contributing to projects with meaningful impact.
Ready to welcome an Android developer who truly understands the nuances of Google’s platform and can contribute to your team in meaningful ways? Discover how Beetroot’s team of Android experts can help bring your project to life.
Subscribe to blog updates
Get the best new articles in your inbox. Get the lastest content first.
Recent articles from our magazine
Contact Us
Find out how we can help extend your tech team for sustainable growth.