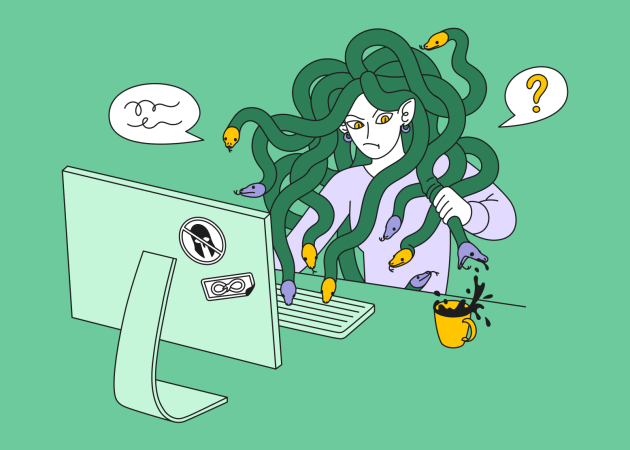
16 Essential Interview Questions for CTOs Hiring Senior Python Developers
Contents
Contents
Finding the right talent is always a strategic advantage. As tech leads and CTOs, we’re not just hiring for skills; we’re seeking individuals who can drive innovation, adapt to changing technologies, and contribute to the culture of excellence that sets your team apart. With its versatility and simplicity, Python has become a cornerstone for many projects, from web applications to data science and machine learning. However, identifying and hiring a senior Python developer who stands out requires a deeper dive.
The questions you ask during an interview are your most powerful tool in this process. They can reveal not only the technical prowess of your candidates but also their problem-solving abilities, approach to collaboration, and capacity for forward-thinking in a landscape that demands constant learning.
This article offers an interview cheat sheet designed to help you uncover the full spectrum of a candidate’s abilities and potential. From technical expertise and scenario-based challenges to soft skills and team orientation, these questions guide you in identifying the senior Python developers who can truly elevate your team.
Let’s dive into the questions that will help you discover not just a candidate who can code but a visionary developer who can navigate the complexities of modern tech environments, contribute to strategic discussions, and push the boundaries of what’s possible with Python.
Technical questions to ask when hiring Python developers
Explore these technical questions designed to assess the depth of expertise of your Python developer candidates.
1. What are Python decorators, and how would you use them?
- Good Answer: A candidate should explain that decorators are a design pattern in Python that allows a user to add new functionality to an existing object without modifying its structure. They might mention using decorators for logging, access control, or memoization.
- Red Flags: Vague or incorrect understanding of decorators, such as confusing them with Java annotations or not being able to provide practical examples.
2. Can you explain the difference between deep and shallow copy in Python?
- Good Answer: Expect a clear explanation that a shallow copy constructs a new compound object and then inserts references into it to the objects found in the original. In contrast, a deep copy constructs a new compound object and then, recursively, inserts copies into it of the objects found in the original.
- Red Flags: Confusion between the two or a lack of understanding of how copying affects mutable and immutable objects.
3. In managing a large-scale Python application, how do you design and implement a comprehensive error handling and logging system to enhance its reliability and maintainability? Please include any advanced tools or practices you utilize.
- Good Answer: A strong candidate would elaborate on utilizing Python’s built-in `logging` module, detailing how to configure loggers, handlers, and formatters to capture application logs effectively. They should discuss the strategic use of `try`, `except`, `else`, and `finally` blocks for robust error handling, emphasizing the importance of catching specific exceptions to prevent the application from crashing and to log useful error information. The inclusion of advanced tools like `Sentry` for real-time error tracking and alerting is a significant plus, as it demonstrates an understanding of modern application monitoring practices. Candidates might also mention integrating logging with application performance monitoring (APM) tools to correlate logs with performance metrics. Discussing the setup of log aggregation solutions (e.g., ELK stack or Splunk) for centralized logging in distributed systems would indicate a comprehensive approach. Additionally, explaining the practice of defining log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) to categorize the severity of log messages and using these logs for debugging and performance monitoring shows a nuanced understanding of logging as a tool for maintaining application health.
- Red Flags: A superficial understanding of error handling and logging, such as relying solely on basic `print` statements for debugging or a lack of familiarity with any external logging or monitoring tools beyond the standard library. Not recognizing the importance of catching and logging specific exceptions or having no strategy for log management and analysis in a large-scale application context indicates a candidate might struggle with maintaining application reliability and diagnosability at scale.
4. What are the key features of Python 3.10+ (or the latest version they are familiar with) that you find most beneficial?
- Good Answer: Look for specific features such as assignment expressions (the “walrus operator”), positional-only parameters, or improved typing extensions. The candidate should explain how these features benefit coding practices.
- Red Flag: Unable to name any new features or improvements in recent Python versions, indicating a lack of continuous learning.
5. Explain the GIL (Global Interpreter Lock) in Python. How does it affect multithreading, and how can you mitigate its impact?
- Good Answer: A good response would include an explanation of how the GIL prevents multiple native threads from executing Python bytecodes at once and strategies to work around it, such as using multiprocessing, concurrent, futures, or integrating with C extensions.
- Red Flag: Misunderstanding the impact of the GIL on performance or suggesting incorrect solutions.
Interview Python Programmers: Technical Scenario-Based Questions
These scenario-based questions challenge Python programmers to demonstrate their practical skills and approach to real-world problems, giving you insight into their hands-on experience and adaptability.
6. Describe your approach to testing in Python. How do you ensure your code is robust and error-free?
- Good Answer: Expect the candidate to talk about a multi-layered testing strategy, including unit tests, integration tests, and end-to-end tests. They should mention using frameworks like unittest, pytest, or nose for writing test cases, and possibly mock for mocking objects. Discussing the importance of test coverage tools like coverage.py to ensure a significant portion of the codebase is tested would also be relevant. Additionally, a good answer might include practices like Test-Driven Development (TDD) or Behavior-Driven Development (BDD) to emphasize the importance of testing in the development lifecycle.
- Red Flag: A lack of familiarity with Python’s testing frameworks or a dismissive attitude toward testing, suggesting it’s not a priority. If a candidate cannot describe a structured approach to testing or the benefits of different types of tests, it may indicate insufficient experience with building reliable, maintainable software.

7. You need to implement a feature that requires data persistence, but the data size is expected to grow significantly. Which database solution would you choose and why?
- Good Answer: Expect a reasoned choice between SQL and NoSQL based on the data’s structure, scalability needs, and consistency requirements. Mention of specific databases (e.g., PostgreSQL, MongoDB) and their advantages in certain scenarios is a plus.
- Red Flag: Choosing a database without considering the application’s specific needs or scalability concerns.
8. You’re tasked with leading the development of a web application that must process real-time data from multiple third-party APIs, each with different response times and reliability. Ensuring the application’s responsiveness and user experience is paramount. How would you approach this challenge, particularly in terms of selecting technologies and designing the system? Are there any specific asynchronous frameworks or libraries you would consider, and why?
- Good Answer: A comprehensive response should demonstrate the candidate’s ability to strategically address the challenge with a deep understanding of asynchronous programming. Key elements of a good answer include:
– Asynchronous Programming: The candidate emphasizes the critical role of asynchronous programming in maintaining application responsiveness, especially when dealing with real-time data from multiple third-party APIs.
– Technology Selection: They discuss evaluating and selecting asynchronous frameworks or libraries, such as Aiohttp, FastAPI, or Sanic, based on factors like project requirements, scalability, ease of use, and the development team’s familiarity. The rationale behind the choice should be clear, whether it’s Aiohttp’s support for high concurrency, FastAPI’s rapid development capabilities and automatic documentation, or Sanic’s ability to handle large volumes of requests.
– System Design Considerations: The candidate outlines additional strategies to optimize the system, such as implementing caching to reduce API load, using message queues for background processing, and establishing robust error handling and retry mechanisms for dealing with API variability.
– Testing and Reliability: They highlight the importance of a thorough testing strategy, including unit tests for asynchronous code and load testing, to ensure the system can handle peak demands and maintain a high level of reliability.
- Red Flags:
– Lack of Specific Frameworks or Libraries: Unable to mention or explain the choice of any asynchronous frameworks or libraries, indicating a lack of knowledge in essential tools for modern Python development.
– Ignoring Asynchronous Programming: Not recognizing the importance of asynchronous programming in the context of handling multiple API requests, suggesting a gap in understanding critical development practices.
– No Mention of Testing or Error Handling: Failing to address how to test asynchronous code or manage errors and latency from third-party APIs, which is crucial for ensuring application reliability and a good user experience.
– Generic or Vague Response: Providing a broad, non-specific answer that doesn’t address the unique challenges presented by the scenario, such as processing real-time data from APIs with varying response times.
9. How do you ensure your Python code remains clean, structured, and well-formatted throughout the development process? Can you discuss the tools and practices you use?
- Good Answer: Candidates should talk about adhering to PEP 8 guidelines for Python code style and mention using tools like `Flake8` for enforcing coding standards, `Black` for automatic code formatting, and `MyPy` for static type checking to catch errors early. They might also discuss the importance of code reviews, using `Git` hooks to automate checks before commits, and integrating these tools into their development workflow to maintain code quality consistently. Mentioning the use of documentation generators like `Sphinx` to keep documentation up to date and aligned with the codebase could also reflect a comprehensive approach to code quality.
- Red Flag: A concerning response would be unfamiliarity with any of the standard Python code quality tools like `Flake8`, `Black`, or `MyPy`. Similarly, not recognizing the importance of coding standards or having no systematic approach to maintaining code quality (e.g., relying solely on manual reviews without tool support) indicates a lack of professionalism or experience in modern Python development practices.
10. Can you describe your approach to ensuring that a Python codebase remains maintainable and scalable as it grows? Include any specific practices, design patterns, or tools you utilize.
- Good Answer: A strong candidate would discuss the importance of following Pythonic conventions and adhering to the PEP 8 style guide for readability. They should mention using modular design principles to keep the codebase organized and maintainable, such as implementing the DRY (Don’t Repeat Yourself) principle and separating concerns through proper use of functions, classes, and modules. The candidate might also talk about leveraging design patterns that are suitable for Python to solve common software design issues in a maintainable way. They could highlight the use of version control systems like Git for managing changes and facilitating collaboration. Additionally, good answers would include the practice of writing comprehensive unit tests with frameworks like `pytest` or `unittest` to ensure code reliability and ease of refactoring. The use of type hints and static type checkers like `MyPy` to catch errors early in a dynamically typed language like Python could also be mentioned as part of a strategy to maintain a clean and scalable codebase.
- Red Flag: Red flags include a lack of mention of any coding standards or conventions, no reference to modular design or design patterns appropriate for Python, and an absence of strategies for testing and quality assurance. If a candidate does not recognize the importance of version control or cannot articulate how they would manage a growing codebase, it suggests they might not be prepared to handle the complexities of a large-scale Python project effectively.
11. Imagine you make a consulting application to reduce the expenses of companies. You have a client base of 10 companies. By the end of a quarter, those companies send a CSV/Excel file with gross revenue and all types of expenses they have plus taxes. With a set of business rules, you need to analyze these files and give an answer within 5 days after you receive them. The average time for processing one file is 21 hours. What technologies and approaches will you use to make it in time?
- Good answer: Tell about ways of parallel processing. Mention that there are several ways to achieve it like using lib multiprocessing or framework as Celery or parallel scalable solution based on AWS Lambda/ECS tasks/Azure Functions etc. with multiple running instances. Compare both approaches and their pros and cons.
- Red flags: Not knowing about any of the approaches or using asynchronous/multithreading without any of the approaches above(asynchronous programming and multithreading can be used here only to speed up file reading).

Python Developer Interview: Questions to Assess Values Match
Uncover the alignment between your organizational values and the team orientation and personal principles of Python developer candidates.
12. Can you give an example of a challenging project you worked on and how you contributed to its success?
- Good Answer: Look for stories highlighting problem-solving skills, collaboration, technical expertise, and the ability to overcome obstacles — the more specific the example, the better.
- Red Flags: Giving a generic answer or failing to demonstrate a significant personal contribution.
13. How do you stay updated with the latest developments in Python and the tech industry?
- Good Answer: Candidates should mention specific resources like conferences, online courses, forums (e.g., Stack Overflow), and reading material (e.g., Python Enhancement Proposals, tech blogs).
- Red Flags: Vague answers or indicating they do not actively seek out new knowledge.
14. Describe a time when you had to give difficult feedback to a colleague. How did you handle it, and what was the outcome?
- Good Answer: Expect an answer that shows empathy and the focus on helping the colleague improve. The outcome should be positive, indicating effective communication.
- Red Flag: Showing discomfort with giving feedback or handling the situation in a way that led to negative outcomes.
15. How do you prioritize tasks when working on multiple projects with tight deadlines?
- Good Answer: Candidates should discuss using prioritization frameworks (e.g., Eisenhower matrix, MoSCoW method), tools (e.g., Trello, JIRA), and communication with stakeholders to align on priorities.
- Red Flag: Lack of a clear strategy for prioritization or suggesting working long hours as the only solution.
16. What is the key to a successful collaboration between development teams and other departments, such as marketing or sales?
- Good Answer: Mention of clear communication, understanding each department’s goals, and using cross-functional meetings or tools to facilitate collaboration. Highlighting the importance of empathy and mutual respect is also good.
- Red Flag: Overlooking the importance of communication and collaboration or showing a lack of respect for non-technical departments.
Python Excellence Made Simple with Beetroot
In the quest for top-tier Python talent, the right questions can illuminate a candidate’s technical prowess, problem-solving abilities, and alignment with your team values. Use the questions above as your toolkit for discerning not just coding skills but the strategic thinking and adaptability essential in the fast-paced tech landscape.
If you’re looking to assemble a Python development team that meets and exceeds your expectations, Beetroot is here to help. Our expertise in building cross-domain tech squads and augmenting your team with highly skilled software engineers ensures that your project is in capable hands, ready to deliver impactful solutions. Contact us today to start building a Python development team that delivers.
Subscribe to blog updates
Get the best new articles in your inbox. Get the lastest content first.
Recent articles from our magazine
Contact Us
Find out how we can help extend your tech team for sustainable growth.